Iterate like a Pro The Complete How-to Guide for Python Iterables (r)
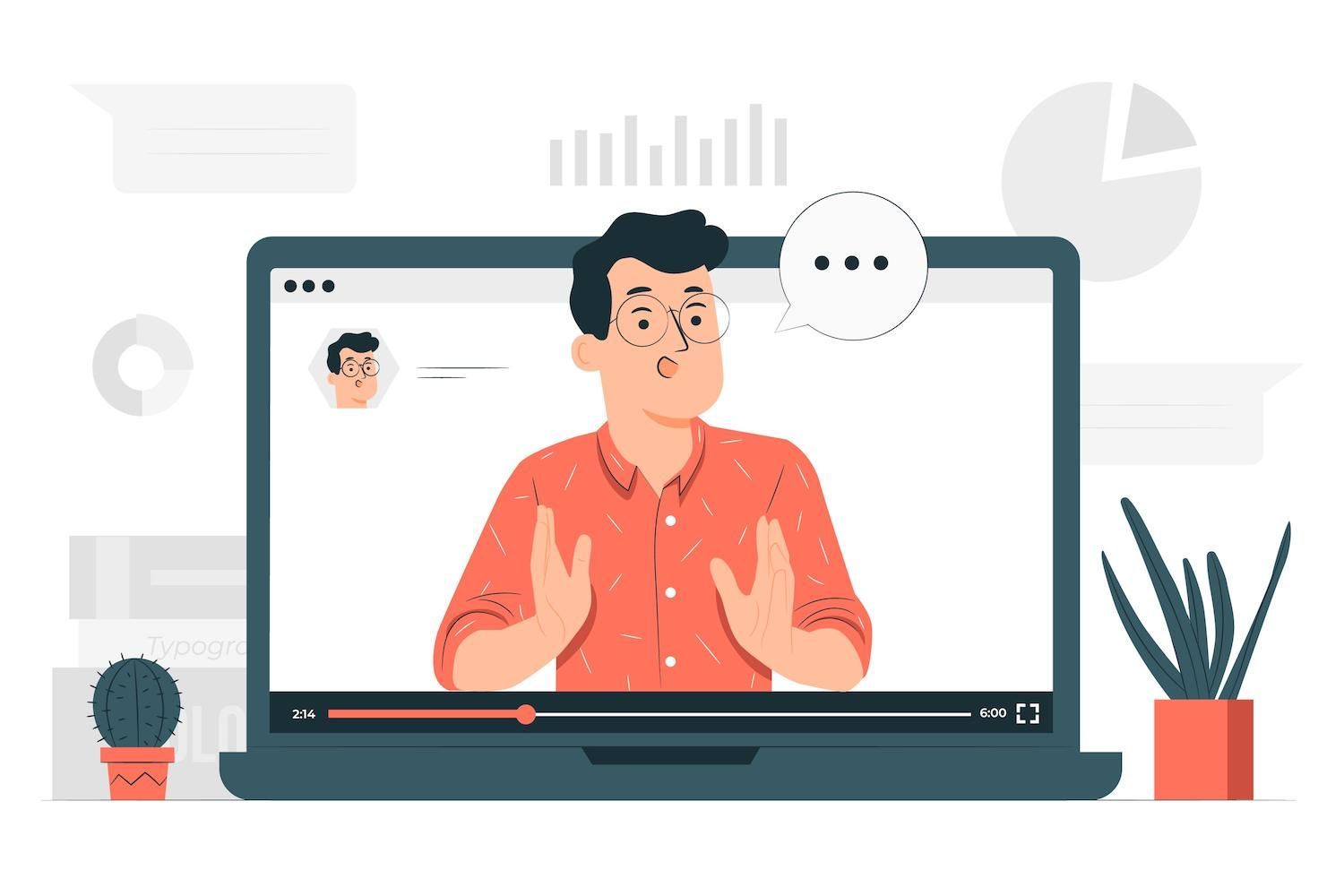
-sidebar-toc>
In Python programming, learning how to code and using iterables efficiently is an essential aspect for you to learn to program effectively. Iterables are objects allow you to loop through. They enable the sequential movements of elements within the boundaries of their own, making an essential instrument for gaining access to and manipulating elements within objects or data structures.
How do I loop through Python Iterables
In Python is an programming language that permits users to loop through various iterative kinds using a for
loop. This lets you navigate the patterns of operations as well as specific items in lists, sets, and the dictionaries.
1. A List of Items that are Looped
Lists are arranged collections of items that allow easy iteration using an for
loop.
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"] for fruit in fruits_list: print(fruit)
In the code below, fruit
acts as an iterator that is used to cycle through every list element as well as printing them. It is ended after the evaluation of the final element of the list. The code will produce the following output:
Apple Mango Peach Orange Banana
2. Iterating with a Tuple
They're similar to lists but they're immutable. It is possible to iterate over them as you would with lists.
fruits_tuple = ("Apple", "Mango", "Peach", "Orange", "Banana") for fruits in fruits_tupleprint(fruit)
In this instance, the loop that for
loop cycles through the tuple. during each iteration, the value of the variable fruit
change to reflect the values of the current element of the tuple. This code will produce an output like this:
Apple Mango Peach Orange Banana
3. Looping Through Sets
Sets are unsorted collections of distinct elements. They can be traversed sets using the for
loop.
fruits_set = "Apple", "Mango", "Peach", "Orange", "Banana" for fruit in fruits_set: print(fruit)
In this example in this instance it is the for
loop iterates through the set. But, because the sets are unsorted and not ordered and unsorted, the order in which iterations occur might not be the exact similar to the sequence that elements were given within the set. In each iteration the fruit variables fruit
will be based its values from the element currently in the set. This code will generate an output similar to the one below (the number of elements may be different):
Mango Banana Peach Apple Orange
4. Repetition of Strings
Strings are characters from the same series, which can be looped, one individual character at a time.
string = "" for string char print(char)print(char)
The below code iterates over"" within the text "" and then each character is printed in a separate line. Each time the iteration process is repeated, the variable character
is changed to match the character of the character currently being displayed within the string. This code produces this output:
KN s T
5. The Traversing of an English Dictionary
Making use of the for
loop can be used to utilize the for loop to create the form of lists, sets, or tuples in addition to strings, but this is different with dictionaries because they use key-value pairs for storing things. Dictionarys are an exceptional case for looping, as they are loopable using various methods. The following are some of the ways you can explore a Python dictionary:
- These keys have been altered:
countries_capital = "USA": "Washington D.C.", "Australia": "Canberra", "France": "Paris", "Egypt": "Cairo", "Japan": "Tokyo" for country in countries_capital.keys(): print(country)
This code generates the list of countries_capital
with country names, where they serve as the keys, while their respective capitals constitute the key values. This for
loop goes through the keys of the countries_capital
dictionary, using key() technique. keys()
method. This method produces an object called a view which displays a list of all the keys found in the dictionary. You can easily go through all keys. When that you run the same loop the variable country
will be reverted to the values of the in use key. The code produces the following outputs
USA Australia France Egypt JapanJapan
- The process of transferring value
countries_capital = "USA": "Washington D.C.", "Australia": "Canberra", "France": "Paris", "Egypt": "Cairo", "Japan": "Tokyo" for capital in countries_capital.values(): print(capital)
In the above code that is in the above code, for
loops through the value in the countries_capital
dictionary, using the numbers(). the is the values()
method. The method generates an object with a view of a listing of all the values included in the dictionary. It makes it simple to go through the entire list of possible values. Every time that you loop,, the capital variable capital
will be re-set to most current value on the list. This code should give this output:
Washington D.C. Canberra Paris Cairo TokyoTokyo
- The method of creating key-value pairs
countries_capital = "USA": "Washington D.C.", "Australia": "Canberra", "France": "Paris", "Egypt": "Cairo", "Japan": "Tokyo" for country, capital in countries_capital.items(): print(country, ":", capital)
The above code shows how to loop through the key and value of the countries_capital
dictionary with the items()
method. The items()
method returns an item view that shows an array of key-value tuples in the dictionary. Within the for
loop, every iteration depacks a key/value pair that is currently within the list. The two variables country
capital and capital
share the same key and value, respectively. This code should give the following output:
USA : Washington D.C. Australia : Canberra The city of CanberraFrance : Paris Egypt : Cairo Japan : Tokyo
Advanced Iteration with enumerate() Python Python
Another option to iterate on Python iterables, and then return the index and the value of elements is through iterate(). iterate()
function. Take a look at this code
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"] for index, fruit in enumerate(fruits_list): print(fruit, index)
Here's the output:
Apple 0 Mango 1 Peach 2 Orange 3 Banana 4
The Enumerate
function allows you to define the index that will be to be used as the start point, besides zero
during the iteration process. You can modify the example in the following manner:
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"] for index, fruit in enumerate(fruits_list, start = 2): print(fruit, index)
This output can be described as:
Apple 2 Mango 3 Peach 4 Orange 5 Banana 6
This illustration shows the start index of the enumeration process, enumerate
does use zero-based indexing for the iterable as does native lists. It just adds the starting value of the list to the very first item in the list all the until the end.
What is the best way to implement Python Generators
Generators are specially-designed Python iterables which allow users to build generator objects by not explicitly creating models that are built in, like lists sets, or dictionary sets. Generators are able to produce values as you go by relying on the generator logic.
Generators employ an yield
statement to return generated value each at a. Here's an illustration of an iterable generator
def even_generator(n) Counter = 0 while counter is n when counter %2 == 0:yield counter counter= 1for num in even_generator(20):print(num)
The code provided defines an even_generator
function which generates even numbers that range from the 0
to a specific number
through yield. yield
expression. The loop used for generating these values, and then runs the same process again using the numeral
iterator, which ensures the evaluation of all values within the defined area. The program produces an odd-numbered table that span between to 20.
up to 20
which is according to:
0 2 4 6 8 10 12 14 16 18 20
You can achieve more efficiency by working using generator expressions. For instance it is possible to develop a generator that may also contain loop logic
cube (num * 3 for number within the range(1, 6,)) to print C in cube (print(c) in cubeprint(c)
Here is the place to designate this cube as a variable cube
for the result of a program that determines the value of the cube from 1 - 6. Then, it loops through the values within that range, and release the outcomes of the computation each one following the next. The output of the program will look in the following format:
1 8 27 64 125
How to build custom iterables
Python lets you further customize iterable operations by using iterators. Iterator objects form component of the iterator protocol, with two options available: __iter__()
as well as next()
. The method called __iter__()
method returns an object which is an iterator, whereas the method called __next()
returns the following value of the container that is iterable. Here's an example of iterators using Python:
even_list = [2, 4, 6, 8, 10] my_iterator = iter(even_list) print(next(my_iterator)) # Prints 2 print(next(my_iterator)) # Prints 4 print(next(my_iterator)) # Prints 6
In this instance iter() method, you will use the the iter()
method to create an iterator ( my_iterator
) from the following list. To gain access to every item in the list, you create an iterator object with the next()
method. Because lists are ordered collections, iterators return every element in a sequential fashion.
Customized iterators are great for operations involving large datasets which you are unable to load into memory concurrently. Because memory is costly and is subject to restrictions in space and space restrictions, it's possible to utilize an iterator to handle the data components separately without loading all of the data in memory.
Multiple Functions
Python utilizes functions to navigate the elements of a list. Common list functions are:
sum
gives the sum of an iterable, as long as it's made up of numerical types (integers floating number, point numbers, ints as well as complex numbers)all
Returnstrue
whenever any element in the list is real. If none of the elements are true elements, it returnsthe value False.
.all
returnstrue
in the event that all elements which are able to be iterated have valid. If not, it returnsTrue.
.max
-returns the highest value which can be derived from an iterable collectionmin
-- The minimum value for an iterable collectionlen
Returns the total length for an iterableappend
-- Appends an additional value to the top of the list.
This illustration illustrates these capabilities using this checklist:
even_list = [2, 4, 6, 8, 10] print(sum(even_list)) print(any(even_list)) print(max(even_list)) print(min(even_list)) even_list.append(14) # Add 14 to the end of the list print(even_list) even_list.insert(0, 0) # Insert 0 and specified index [0] print(even_list) print(all(even_list)) # Return true only if ALL elements in the list are true print(len(even_list)) # Print the size of the list
The result is:
30 True10 2[2, 4 6, 8, 10, 14] [0 2, 4 7 8 14] False7
In the previous example Append is the function that is used to append. The
function is used to add the numbers ( 14
) in the middle of the list. Insert function permits you to specify the index for insertion. function insert
function permits specifying the index to insert. So, even_list.insert(0 (0, 0)
inserts 0
at index [0insert[0](0, 0)
. The statement print(all(even_list))
returns false
because there is a 0
value in the list, interpreted as false
. Finally, print(len(even_list))
outputs the length of the iterable.
Advanced Iterable Operations
Python offers advanced capabilities that allow for a more concise process. Listed below are some of these capabilities.
1. List Comprehensions
List comprehensions let you make fresh lists simply by adding actions to every item on existing lists. Here's an illustration
my_numbers = [11, 12, 13, 14, 15, 16, 17, 18, 19, 20] even_number_list = [num for num in my_numbers if num%2 == 0] print(even_number_list)
In this code snippet an array of numbers called my_numbers
can be generated by using numbers ranging between 11
all the way to twenty
. The goal of this list is to make an entirely different list, even_number_list
that contains only even integers. In order to achieve this, use a list comprehension that returns the number of integers that are derived from my_numbers
only when the integer is even. The is
clause contains the logic that returns the even numbers.
The result is:
[12, 14, 16, 18, 20]
2. Zip
The Python zip()
function combines many iterables to create Tuples. Tuples are able to store a range of variables, and they are indefinitely changeable. Learn how to mix iterables using Zip()
:
fruits = ["apple", "orange", "banana"] rating = [1, 2, 3] fruits_rating = zip(rating, fruits) print(list(fruits_rating))
In this example, fruits_rating
pairs each fruit's rating giving one iterable. Its output will be:
[(1, 'apple'), (2, 'orange'), (3, 'banana')]
The code functions as an instrument for rating various fruits. The first list ( fruits
) representing the fruits while another list presents ratings on a scale from one to three.
3. Filter
Another function that is superior, called filter, is a function that takes two arguments that comprise functions and an iterable. The function is applied to all elements of the iterable. It then creates a new iterable containing only those elements for which the function returns a authentic
value. The following example shows the filtering process of an array of numbers within the specified range, returning only those which are:
def is_even(n): return n%2 == 0 nums_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_list = filter(is_even, nums_list) print(list(even_list))
As you can see as in the previous code you begin by creating an algorithm is_even,
that calculates an even number that is passed to it. You then create an integer list that is between 1 and 10-- the list of nums
. In the end, you design a new list, even_list
, which uses it's filter()
function to use the process you've specified on the previous list to display only the parts of the list which are. It is the result:
[2, 4, 6, 8, 10]
4. Map
As with filter()
, Python's map()
function takes an iterable along with an argument in the form of arguments. However, instead of returning all elements from the previous iterable, it generates a brand new iterable that contains the results of the method that was applied to the elements in the previous iterable. If you want to create a quadrupled list of numbers, take advantage of this map()
function:
my_list = [2, 4, 6, 8, 10] square_numbers = map(lambda x: x ** 2, my_list) print(list(square_numbers))
In this code, x
is the iterator which traverses the list before transforming it by using the algorithm of squares. Map() function mapping()
function executes the process using the list's original argument, and then the mapping function. The output is as follows:
[4, 16, 36, 64, 100]
5. Sorted
The sort
function arranges all the elements of an iterable in a way that is specific (ascending or decreasing) and gives the result as an array. It can take in the three maximum parametersthe following: iterable
, reverse
(optional) in addition to iterable, reverse (optional), and the key
(optional). It then, reverse
defaults to False
when you set it to the value True.
and the components are arranged in ascending the order they appear in. key
is a function which calculates a number in order to determine the order in which the element. The value is iterable element, which defaults to the value None
.
Here's an example of how to apply the Sort
function for different iterables:
# set py_set = 'e', 'a', 'u', 'o', 'i' print(sorted(py_set, reverse=True)) # dictionary py_dict = 'e': 1, 'a': 2, 'u': 3, 'o': 4, 'i': 5 print(sorted(py_dict, reverse=True)) # frozen set frozen_set = frozenset(('e', 'a', 'u', 'o', 'i')) print(sorted(frozen_set, reverse=True)) # string string = "" print(sorted(string)) # list py_list = ['a', 'e', 'i', 'o', 'u'] print(py_list)
You will get the following output:
['u', 'o', 'i', 'e', 'a'] ['u', 'o', 'i', 'e', 'a'] ['u', 'o', 'i', 'e', 'a'] ['a', 'i', 'k', 'n', 's', 't'] ['a', 'e', 'i', 'o', 'u']
How to deal with errors and Edge Cases Iterables
Edge cases are often encountered in a variety of programming situations, and you should anticipate them in iterables. We'll look at a few possible scenarios that you could come across.
1. Incomplete Iterables
There are times with an empty iterable but a program logic attempts to handle it. You can address this by programming to prevent inefficiencies. This is an example of using an If-not
expression to ensure that an item is not empty.
fruits_list = [] if the list is not fruit-related It is not,print("The list is not complete")
It's the result.
The table is not empty
2. Nested Iterables
Python is also able provide nested Iterables that contain iterable objects that have other iterables within their. For instance, you can build lists of foods that include various categories of food items that are nested like vegetables, meats and grains. Let's look at how you can model the scenario by using nested iterables
food_list = [["kale", "broccoli", "ginger"], ["beef", "chicken", "tuna"], ["barley", "oats", "corn"]] for inner_list in food list:to list food items in the inner list:print(food)
In the code above, you can see that in the code above, you will see that food_list
variable is comprised of three nested lists, representing diverse food groups. The loop that iterates through ( for inner_list in food_list
) runs over the primary list, and inside this loop ( for food in the inner list:
) iterates through each nested list, printing each food item. Its output can be described as follows:
Kale broccoligingerchicken tunabarley oats,corn
3. Exception Handling
In Python iterables, they also provide exception handling operations. In this case, for instance, you could browse through a list and come across an index error
. This means that you're trying reference to something which is outside of the boundaries of the iterable. How do you deal with this kind of error? Use an try-to-exclude
block?
fruits_list = ["apple", "mango", "orange", "pear"] try: print(fruits_list[5]) except IndexError: print("The index specified is out of range. ")
The following code shows that the list of fruits
iterable consists of five elements with indices of five
to five
in the list. The phrase you want to test check
phrase contains the print
function that tries to display the value on five in the iterable. five
within the iterable, but doesn't exist. This executes the except clause and then returns an error message. The console returns the error:
The index you are looking for does not fall within the range.
Summary
Learn how to iterate with Python is essential to write proficient and easy-to-read programming. Understanding the various ways to create different types of of data structures using comprehensions, generators, using built-in functions, makes you a competent Python programmer.
Have we missed anything from the guide? Let us know about it in the comment section!
Jeremy Holcombe
The Content and Marketing Editor at , WordPress Web Developer, as well as Content writer. Aside from everything WordPress I love playing golf and at the beach, and watching movies. Additionally, I'm tall. issues ;).
This post was first seen on here