How to add Rate Restrictions to an API inside the Laravel Application - (r)
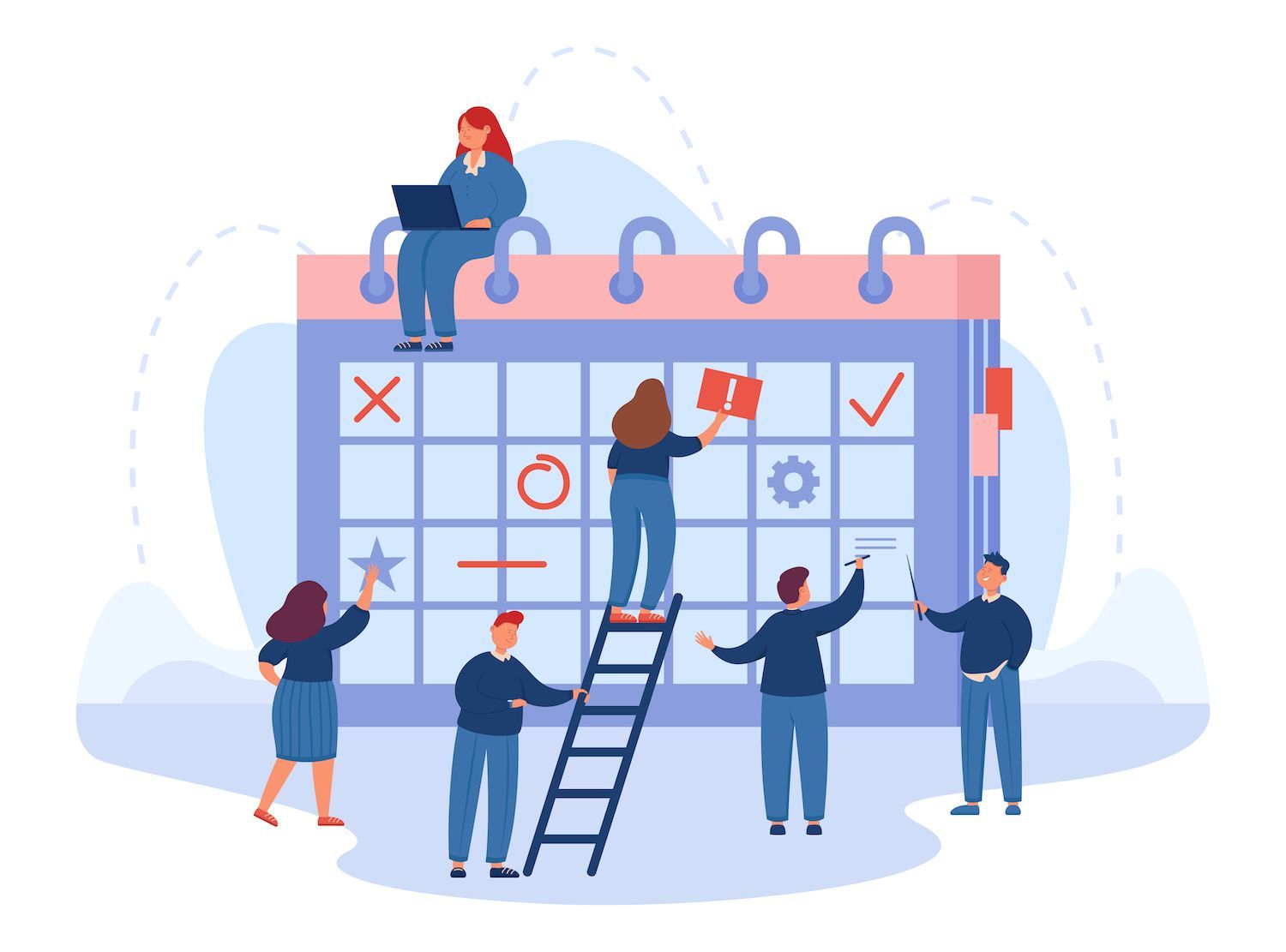
-sidebar-toc>
Restricting usage is crucial to guard the resources of your app or website from misuse or excessive use. This could be due to an intentional human error, bot-driven attacks, or simply a misidentified attack, a misuse of resources could hinder the access you have granted to your app and introduce severe vulnerability.
This article will explain the ways to include rates limiting within an API in the Laravel application.
Throttle Your API Traffic in Laravel
The principle behind rate-limiting was designed to restrict use of the application's resources. It has a variety of uses, it is particularly useful for APIs that are public in large, high-performance platforms. It makes sure that all authorized users are able to access all resources available to the system.
Reducing the amount of data transfer is essential to ensure security in cost management as well as general security for the overall stability of your system. This can prevent the occurrence of attacks based on requests, such as distributed denial-of-service (DDoS) attack. This type of attack involves repeated sending to overload the system and cause disruption to the access to websites or the web server.
There's an array of methods to setting rates limits. You can use variables that identify the user to decide who can access your application and at what frequency. Some common variables include:
- IP address Implementation of rates that are dependent on IP addresses allow users to limit the amount of requests per address. This can be particularly helpful in situations where the user is able to gain access to an application without a login.
- API Key Access restrictions via API keys entails offering the user with an API key that's been generated, and setting limit on the rate of access per key basis. This method allows you to also assign different levels of access to the created API keys.
- Client ID It is possible to create a Client ID which users can embed in the header or body of API request. It allows you to define for each ID access levels, ensuring that each client does not have the capability of monopolizing the resources available to it.
Laravel Middleware
How do you best to implement rate limits?
This tutorial makes use of an API for mini libraries that is built upon the Laravel 10 framework to demonstrate how to utilize Laravel Throttle. The starter project sample contains the basic create read, update and remove (CRUD) implementations required to manage books in the range, along with two other methods of demonstrating certain limitations to rates.
The conditions
This guide assumes you're comfortable with the fundamentals of API development with Laravel. You must have the following skills:
- PHP 8.2, Composer, and Laravel is installed and set-up locally on your device
- An account with a user on GitHub, GitLab and Bitbucket for pushing your software
You also utilize the My to set up and then launch the API. Follow the included project template before examining the output of the entire program code.
Laravel Application Installation
- In the beginning, copy the design template..
- Create a .env file in the project's root directory and duplicate the contents in .env.example into it.
- After that, finish your installation by following the steps to install app dependencies and create the application key.
composer install php artisan key:generate
If this command fails to instantly add the app keys to the .env file, execute the php artisan key:generate command with --show
and then copy the generated key and add the key to your .env file as the key value in APP_KEY
.
- Once the dependencies installation and the key generation for application is done, open the application with the following option
php artisan serve
This command starts the application and makes it accessible via the browser at https://127.0.0.1:8000
.
- Go to the URL and confirm that the Laravel welcome page appears:
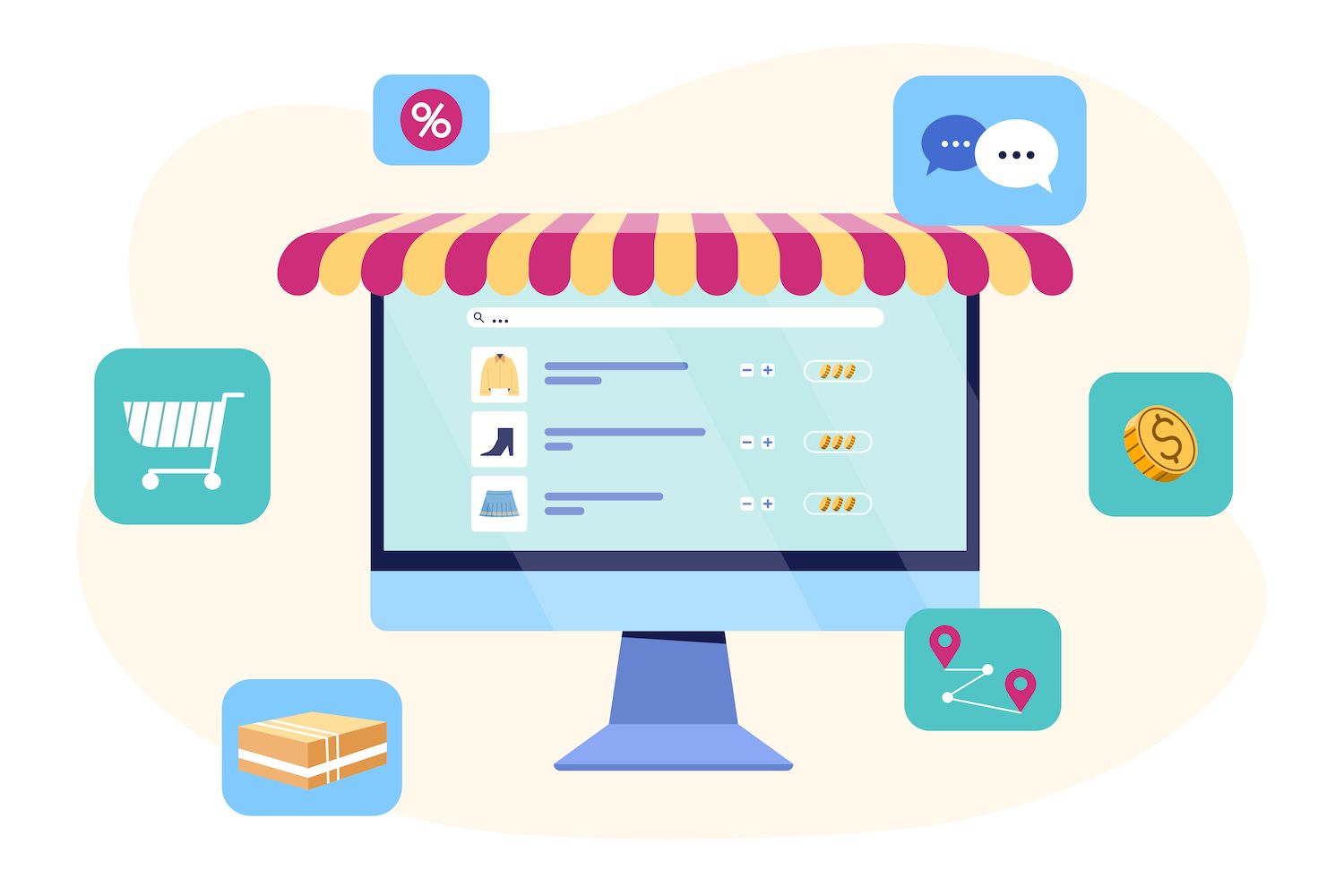
Configuring Databases
Set up and configure the database for application in My.
- Log into the My Account dashboard, then select Add service. "Add service" button:
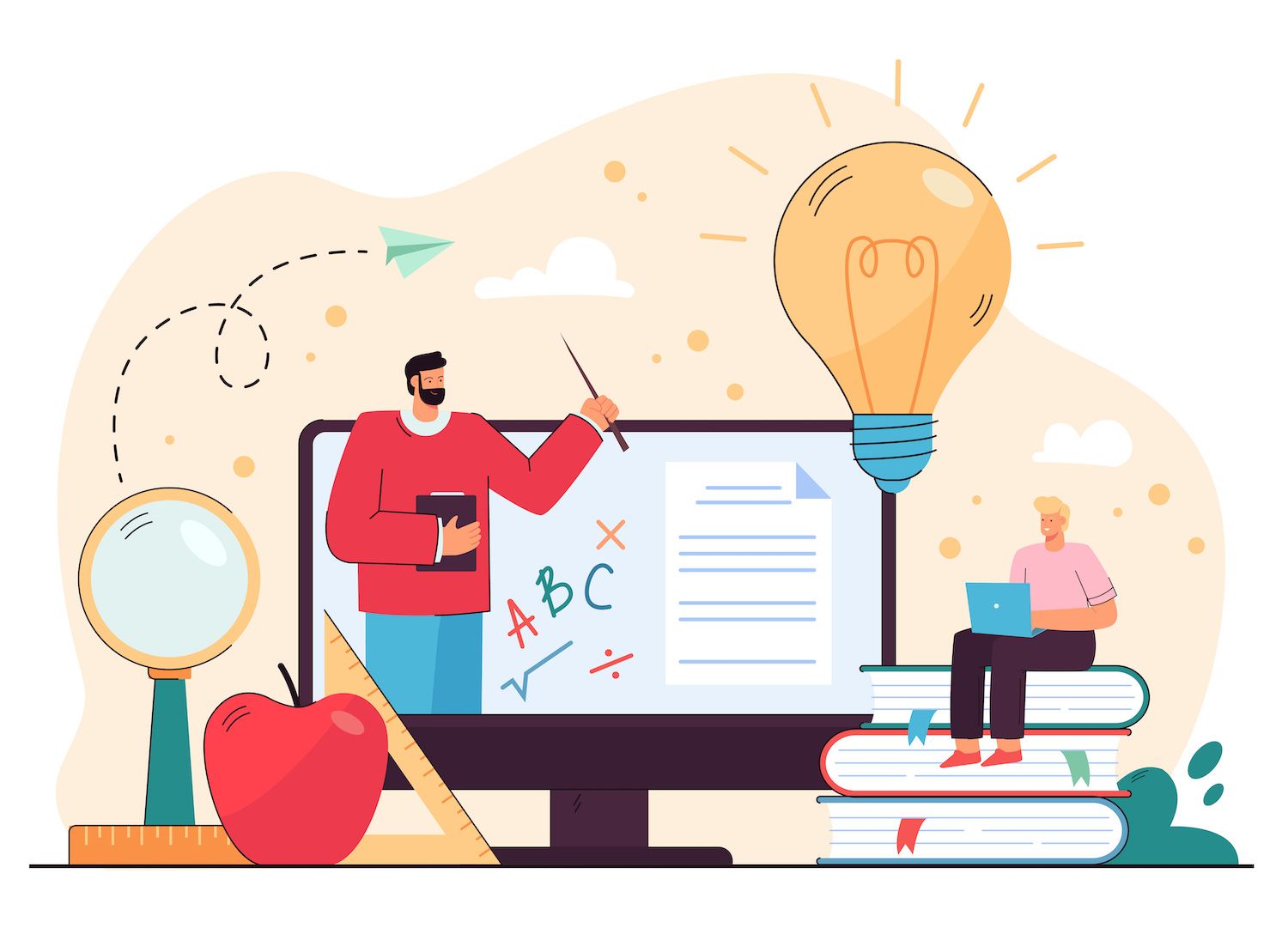
- In the Add Service list, click "Database" and set the parameters that will start your database instance:
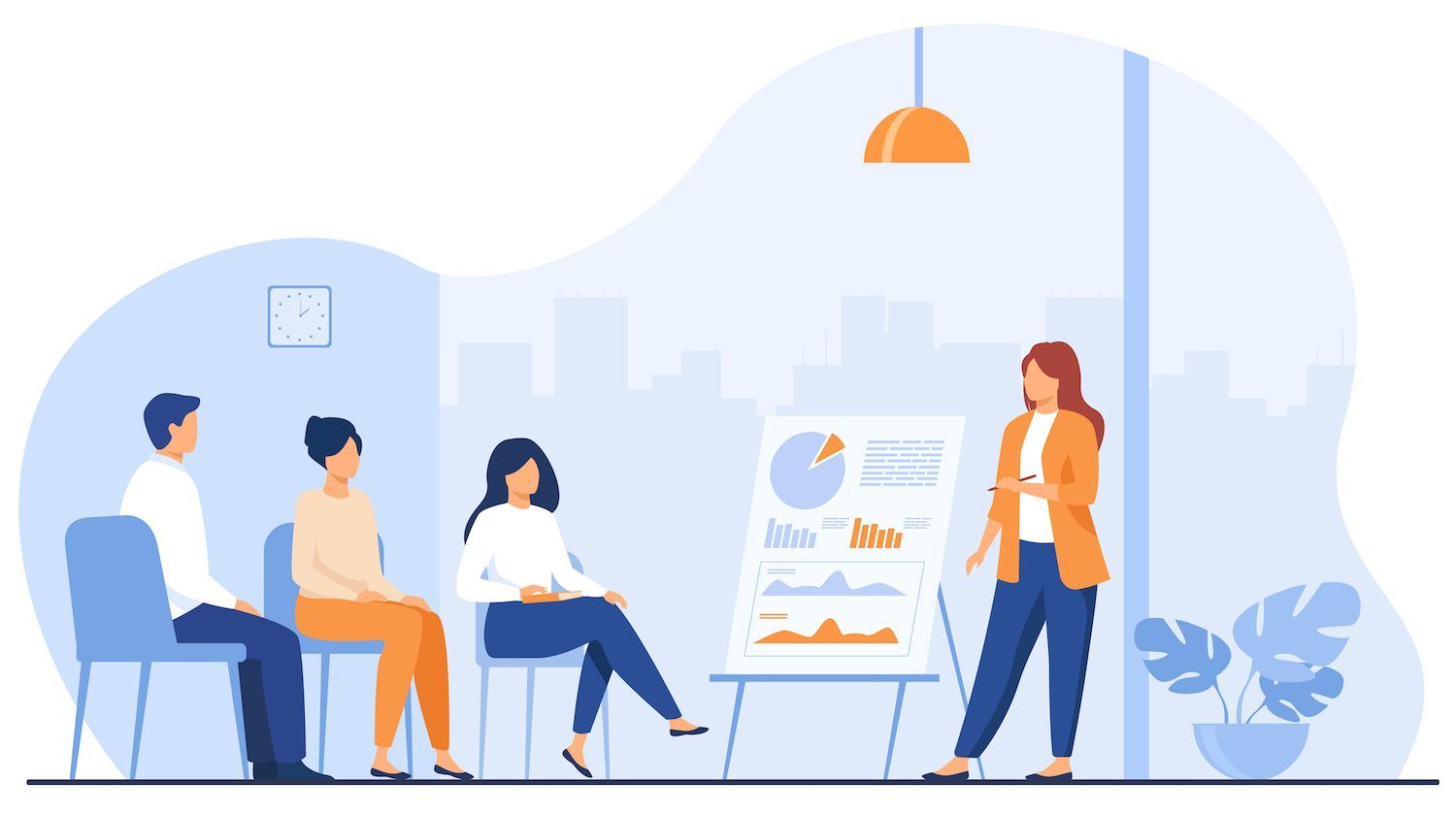
This tutorial uses MariaDB however you can select one of the other supported Laravel databases which offer.
- When you've finalized your database details, click on the Proceed button to finalize the procedure.
Databases provisioned on have connections to external and internal. Best practice is to connect internal parameters for applications hosted in the same account as additionally external connection parameters that allow you to connect externally. Thus, make use of's the credentials of an external database in your application.
- Transfer and edit the app's database .env credentials with external credentials like illustrated in the following screenshot:
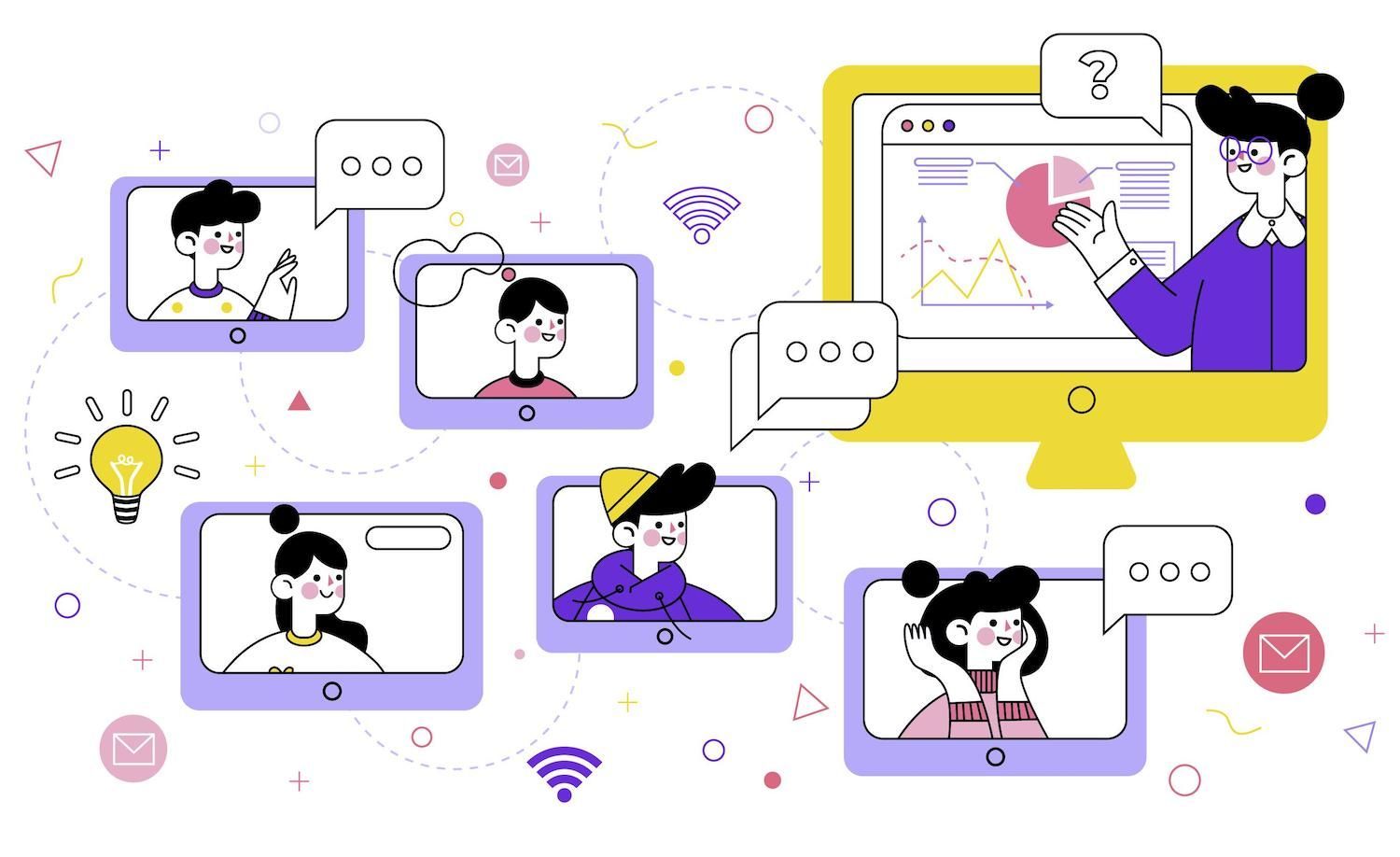
DB_CONNECTION=mysql DB_HOST=your_host_name DB_PORT=your_port DB_DATABASE=your_database_info DB_USERNAME=your_username DB_PASSWORD=your_password
- Once you have entered the credentials to the database, check your connection by using Database migration using these commands:
php artisan migrate
If everything is functioning properly, you should get exactly the same result as in below.
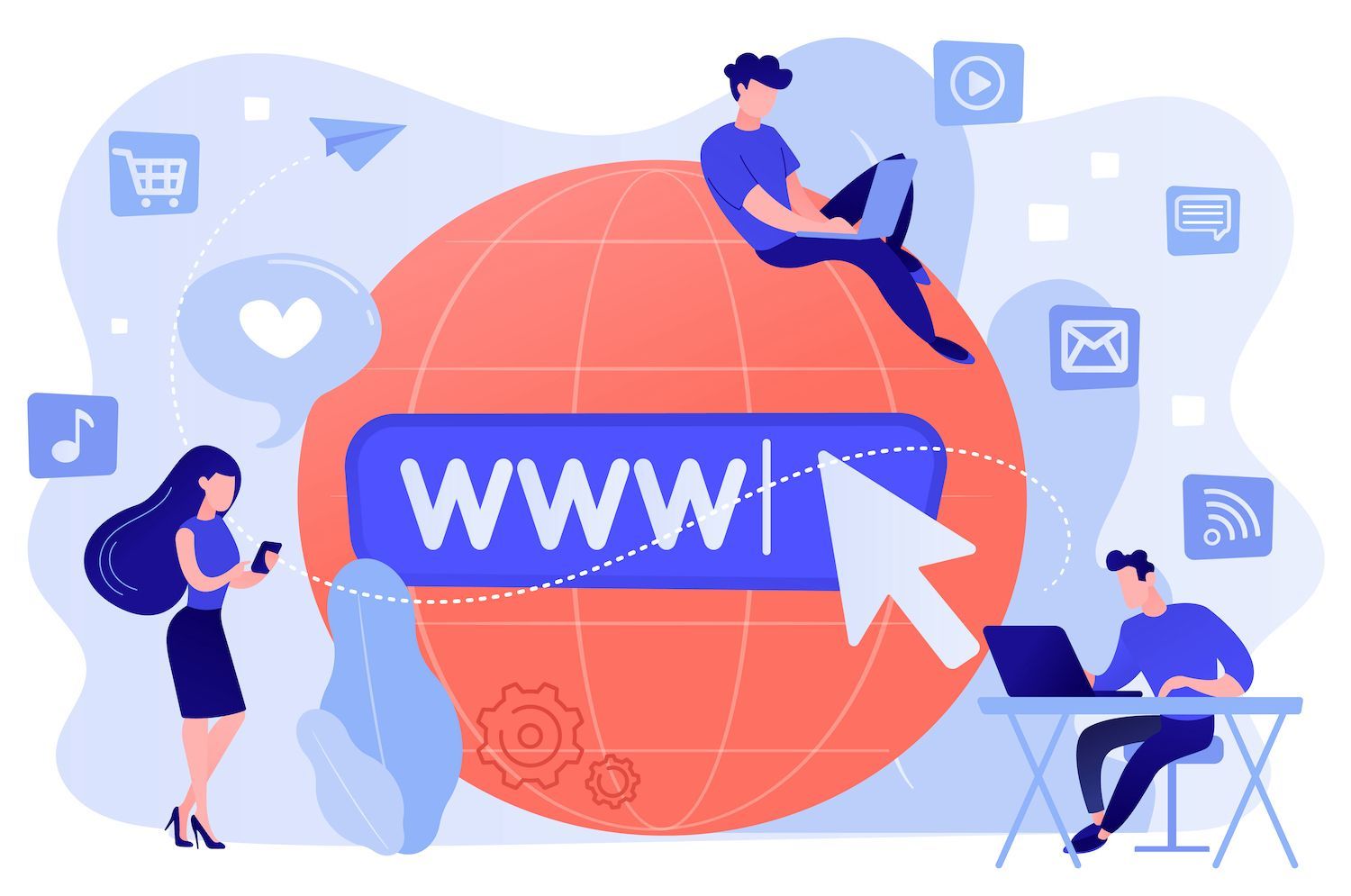
- Then, select the next option to display all the apps routes. There are also routes that have been implemented.
php artisan route:list
It is now possible to access the API ends that are accessible:
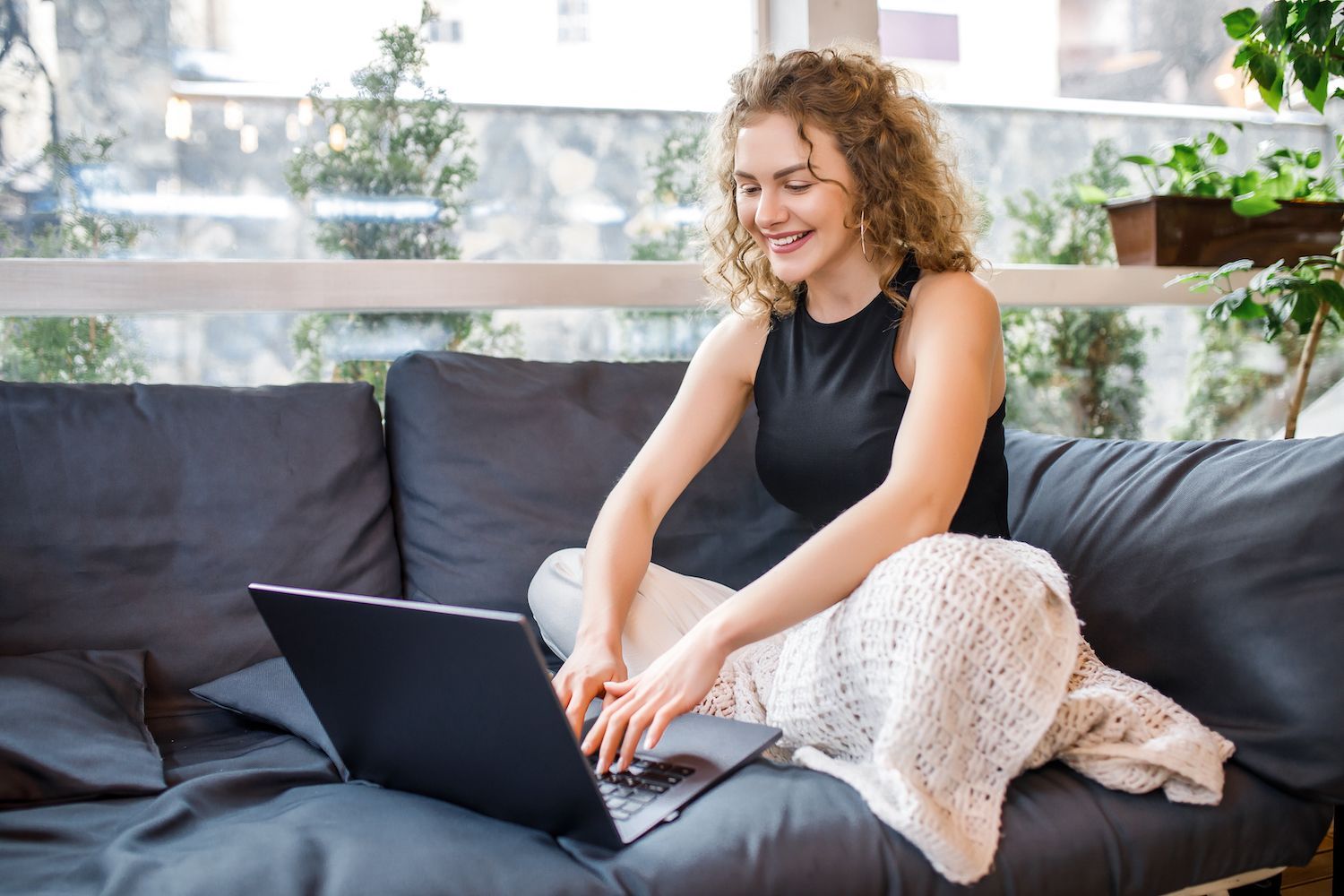
- Start the application and confirm that everything is still working. Test these endspoints with a terminal application such as Postman or CURL.
What is the best way to limit your rate within the Laravel Application
- Installation of the Laravel Throttle package using the following instruction:
composer require "graham-campbell/throttle:^10.0"
- It is also possible to alter the Laravel Throttle configurations through the publication of the
vendor configuration
file.
php artisan vendor:publish --provider="GrahamCampbell\Throttle\ThrottleServiceProvider"
How do you block IP addresses?
Another method of rate-limiting lets you block access for an IP group of addresses.
- In the beginning you will need to create the middleware needed:
php artisan make:middleware RestrictMiddleware
- Next, open the created app/Http/Middleware/RestrictMiddleware.php middleware file and replace the code in the
handle
function with the snippet below. Make sure you addUse App
in the list of imports on the very top of your middleware file.
$restrictedIps = ['127.0.0.1', '102.129.158.0']; if(in_array($request->ip(), $restrictedIps)) App::abort(403, 'Request forbidden'); return $next($request);
- Inside the app/Http/Kernel.php Create an Middleware alias by updating to the
middlewareAliases
array as follows:
protected $middlewareAliases = [ . . . 'custom.restrict' => \App\Http\Middleware\RestrictMiddleware::class, ];
- After that, you can apply the middleware on the
route/restricted
to the routes/api.php file as the following and try:
Route::middleware(['custom.restrict'])->group(function () Route::get('/restricted-route', [BookController::class, 'getBooks']); );
When working correctly, this middleware blocks all requests from IP addresses within the array: $restrictedIps
array. This includes 127.0.0.1
and 102.129.158.0
. If you try to reach these IPs, they will return an error message of 403 unaccepted response. The following illustration:
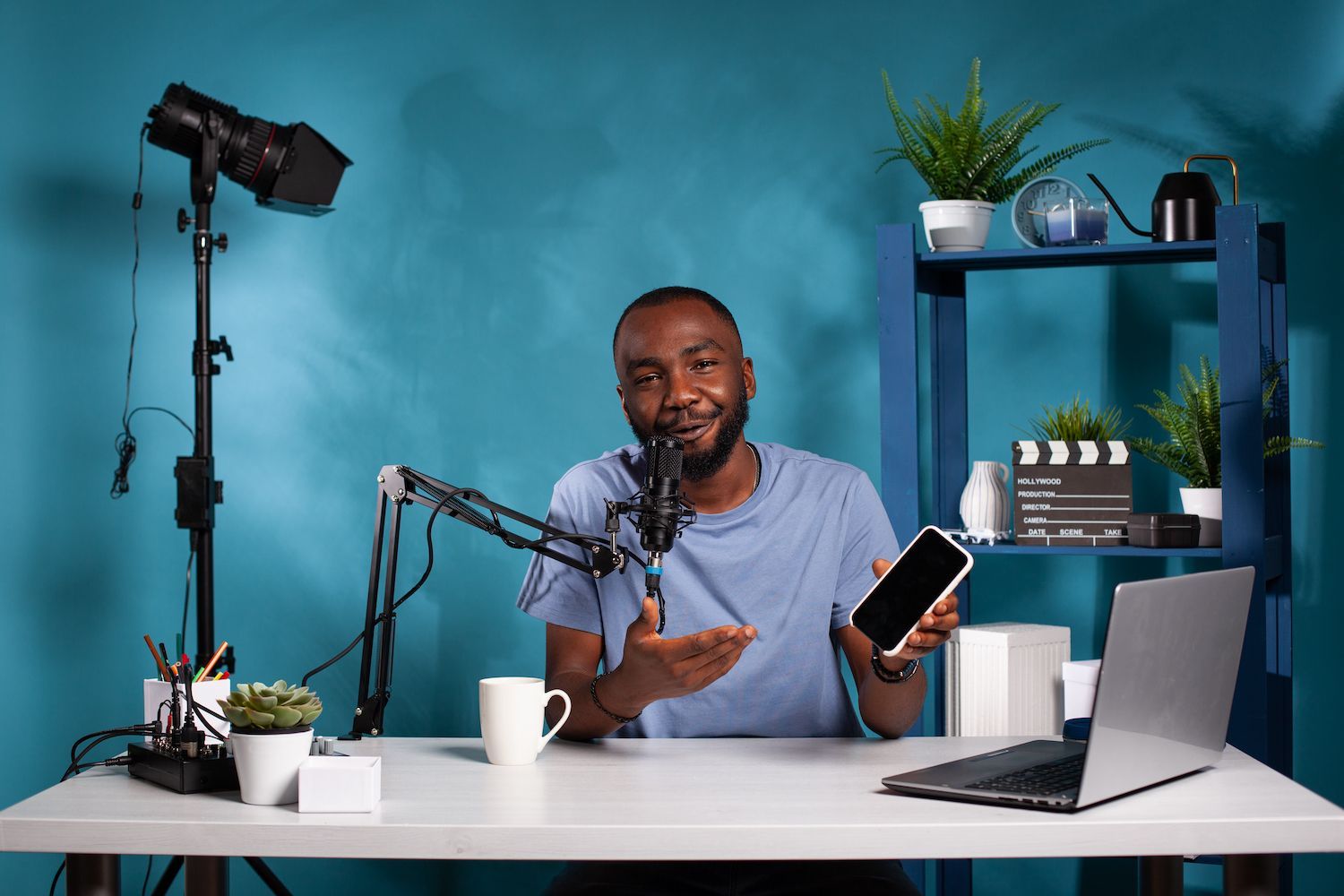
How to throttle requests via IP Address
In the next step, you'll rate the each request based on your IP address.
- Install this Throttle middleware onto the
the /book
connection'sPATCH
ANDPATCH
routes. routes/api.php:
Route::middleware(['throttle:minute'])->group(function () Route::get('/book', [BookController::class, 'getBooks']); ); Route::middleware(['throttle:5,1'])->group(function () Route::patch('/book', [BookController::class, 'updateBook']); );
- You must also update the
configureRateLimiting
function in the app/Providers/RouteServiceProvider file with the middleware you added to the above routes.
... RateLimiter::for('minute', function (Request $request) return Limit::perMinute(5)->by($request->ip()); );
The request limit is set for the book The book
ends at 5 minutes. This is illustrated in the following.
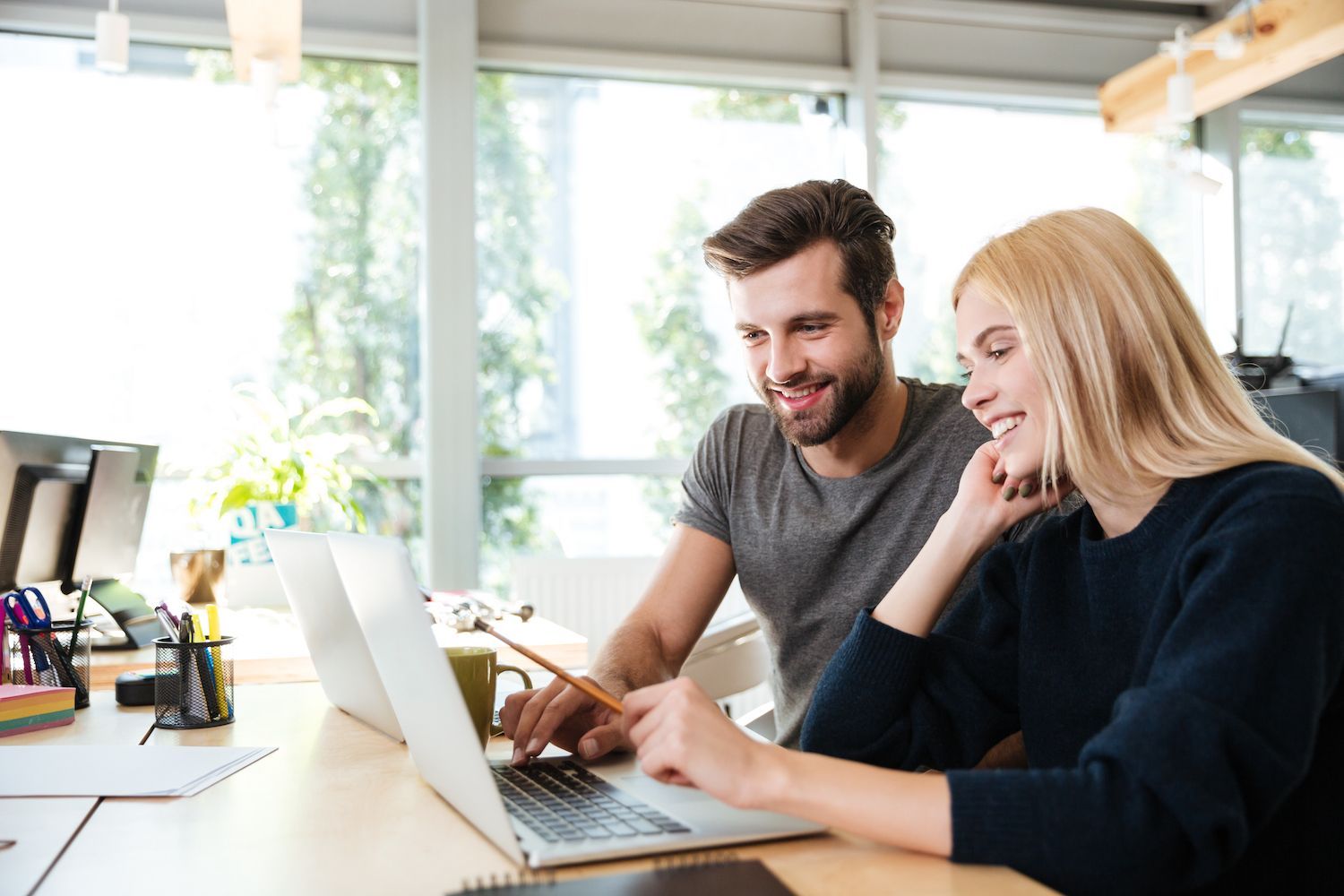
What to throttle based on the User ID and Sessions
- To rate limit using
user_id
andsession
parameters, update theconfigureRateLimiting
function in the app/Providers/RouteServiceProvider file with the following additional limiters and variables:
... RateLimiter::for('user', function (Request $request) return Limit::perMinute(10)->by($request->user()?->id ? : $request->ip()); ); RateLimiter::for('session', function (Request $request) return Limit::perMinute(15)->by($request->session()->get('key') ? : $request->ip()); );
- Then, you can apply this code to
ID /book/GET
as well asthe book POST
routes of theroutes/api.php
file:
Route::middleware(['throttle:user'])->group(function () Route::get('/book/id', [BookController::class, 'getBook']); ); Route::middleware(['throttle:session'])->group(function () Route::post('/book', [BookController::class, 'createBook']); );
This limit code restricts requests that utilize the user_id
and session session
as well as session.
Alternative Methods for Throttling
Laravel Throttle features several other techniques for ensuring greater control over your rate-limiting app. The methods are:
attempt
-- Hits the limit it reaches, increases the count of hits and returns a boolean that indicates whether the limit for hits set is over.hit
hit -- hits the Throttle, increases the number of hits. It returnsthe amount
to enable another (optional) method to call.clear
resets the throttle number all the way back to zero. It then returns$this
to make another call, if you'd like to.count
-It returns the total number of hits to the Throttle.test
returns an objctan that indicates whether the Throttle hit limit was exceeded.
- For a test of rate-limiting techniques, you can create a middleware application named CustomMiddleware with the below command:
php artisan make:middleware CustomMiddleware
- Then, add the following import files to the newly created middleware file in app/Http/Middleware/CustomMiddleware.php:
use GrahamCampbell\Throttle\Facades\Throttle; use App;
- Following that, alter the contents of handle method using the following code: Replace
the handle
method using the following code sample:
$throttler = Throttle::get($request, 5, 1); Throttle::attempt($request); if(!$throttler->check()) App::abort(429, 'Too many requests'); return $next($request);
- Within the app/Http/Kernel.php file, set up an alias of this middleware app, by changing the middlewareAliases array.
middlewareAliases
array in the following manner.
protected $middlewareAliases = [ . . . 'custom.throttle' => \App\Http\Middleware\CustomMiddleware::class, ];
- Then, you are able to use this middleware on the
customized-route
within your routes/api.php file:
Route::middleware(['custom.throttle'])->group(function () Route::get('/custom-route', [BookController::class, 'getBooks']); );
The middleware customized that was just recently put in place will be able to determine if the limit on throttle has been exceeded by employing the check/code technique. If the limit has been overridden, it will give a 429 error. In other cases, it will allow the request to go on.
How can the application be deployed onto the Server
After you've learned how to set up rate limits in a Laravel application, be sure you deploy your application to the server in order for it to be accessible to the world.
- On your dashboard, select from the "Add Services" button and then select the program in the menu. Connect your Git account with your existing account and choose the right repository to be deployed.
- In the Basic Info, name the application and choose the location you prefer for your data center. Also, ensure you added the required environment variables for your application. They're the same as the ones in your individual .env file: app_key,
app_key
along with the database configuration variables.
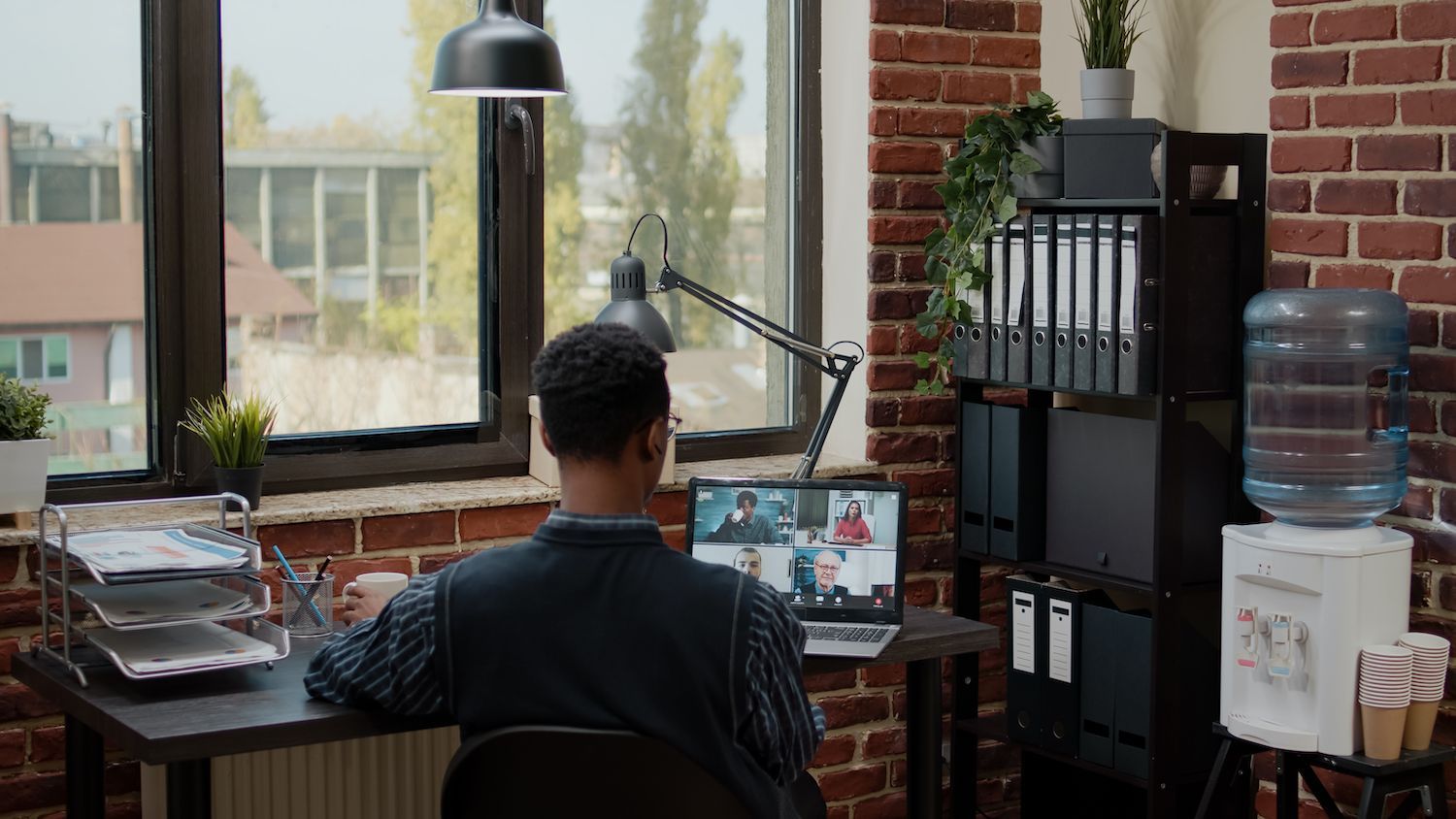
- Use The Keep button to pick the construct environment variables. It is possible to leave the default values because the auto-fill function fills every necessary parameter.
- On the Processes tab there is the option to leave the default values or choose a different name for the procedure. You can also pick the pod or instance sizes in the tab.
- In the final step, the tab for payment tab offers a listing of options. Pick the payment method that you'd like to make the transaction.
- Once you have done that, head to and click the Application tab to view the list of applications that have been deployed.
- Select the name of the application to get more information on its use, like the one below. Use the URL to access it.
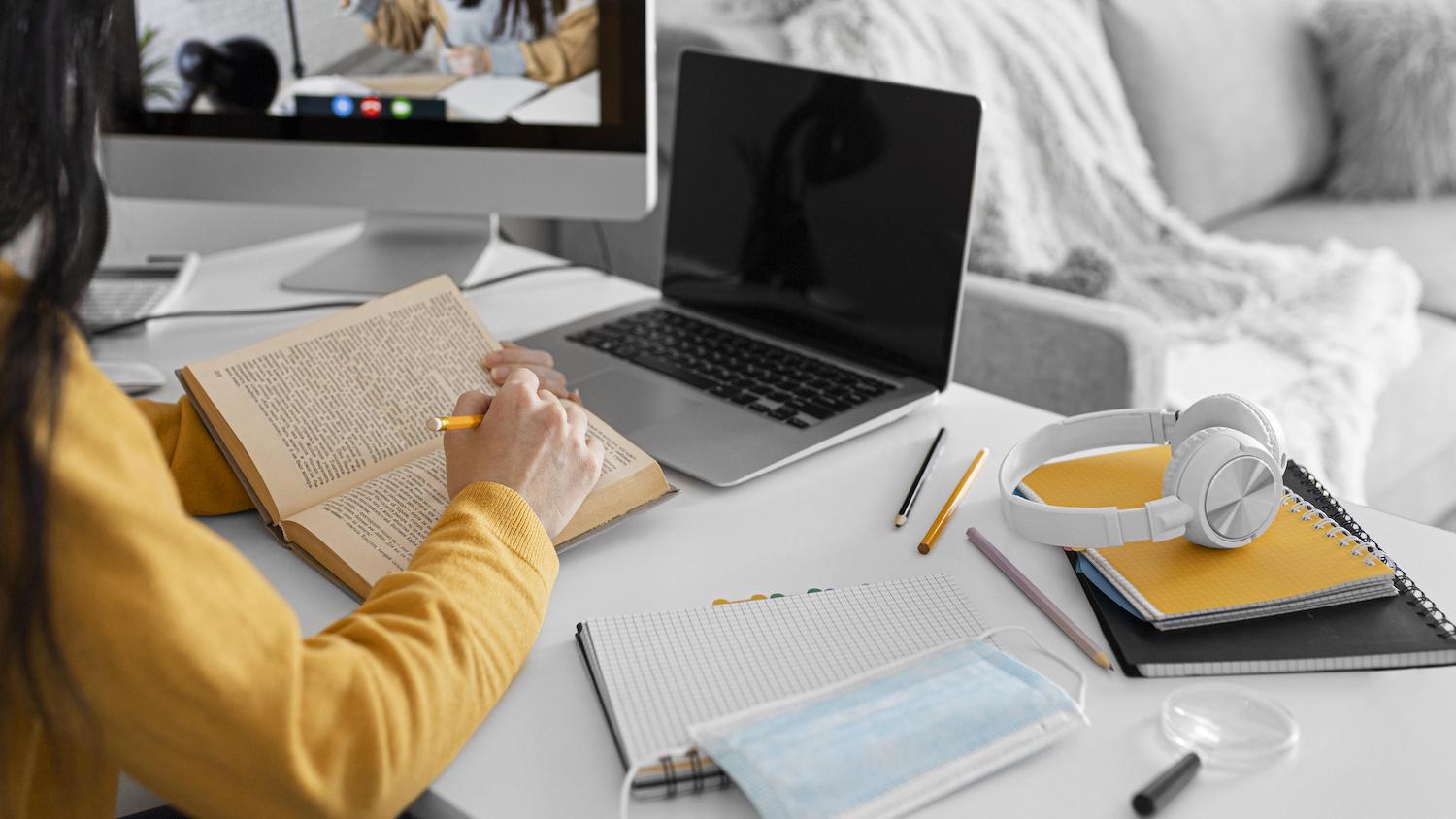
What can you do to test the App
- To test the application on your local computer, you may utilize the
PHP artisan Serve
command.
This command makes your application browser accessible at http://localhost:8000
. It is possible to test the API endpoints you have created rate-limiting on this page, by repeatedly calling to invoke the function of rate limiting.
The server displays it with the access forbidden response because you haven't provided the necessary configuration details on the best way to host your application. Add these details now.
- Create the
.htaccess
file in the root directory of your app and then insert the following code in the file:
RewriteEngine on RewriteRule(. *)$ public/$1 [L]
- Make these changes available to the public on GitHub as well as auto-deployments to carry out the changes.
- Open the application via the link provided, and ensure you're at the Laravel welcome page.
It is now possible to check the API endpoints you have implemented rate-limiting with Postman with a series of requests until you've reached your set limit. The response is an error message that reads 4029: To many requests response after exceeding the limit.
Summary
Utilizing features that limit the rate of use in this Laravel API allows you to regulate the way people use the application's resources. This helps to ensure that users enjoy a safe and reliable experience without under and over-spending. This also helps ensure that your foundational infrastructure is effective and functional.
Marcia Ramos
I'm the editor's team lead at . I'm a fan of open source and love programming. More than seven decades of writing technical documents experience as well as editing experience in the field of technology, I love collaborating with others in writing simple, clear documents and to improve workflows.
Article was first seen on here