A Comprehensive Guide To Laravel Authentication
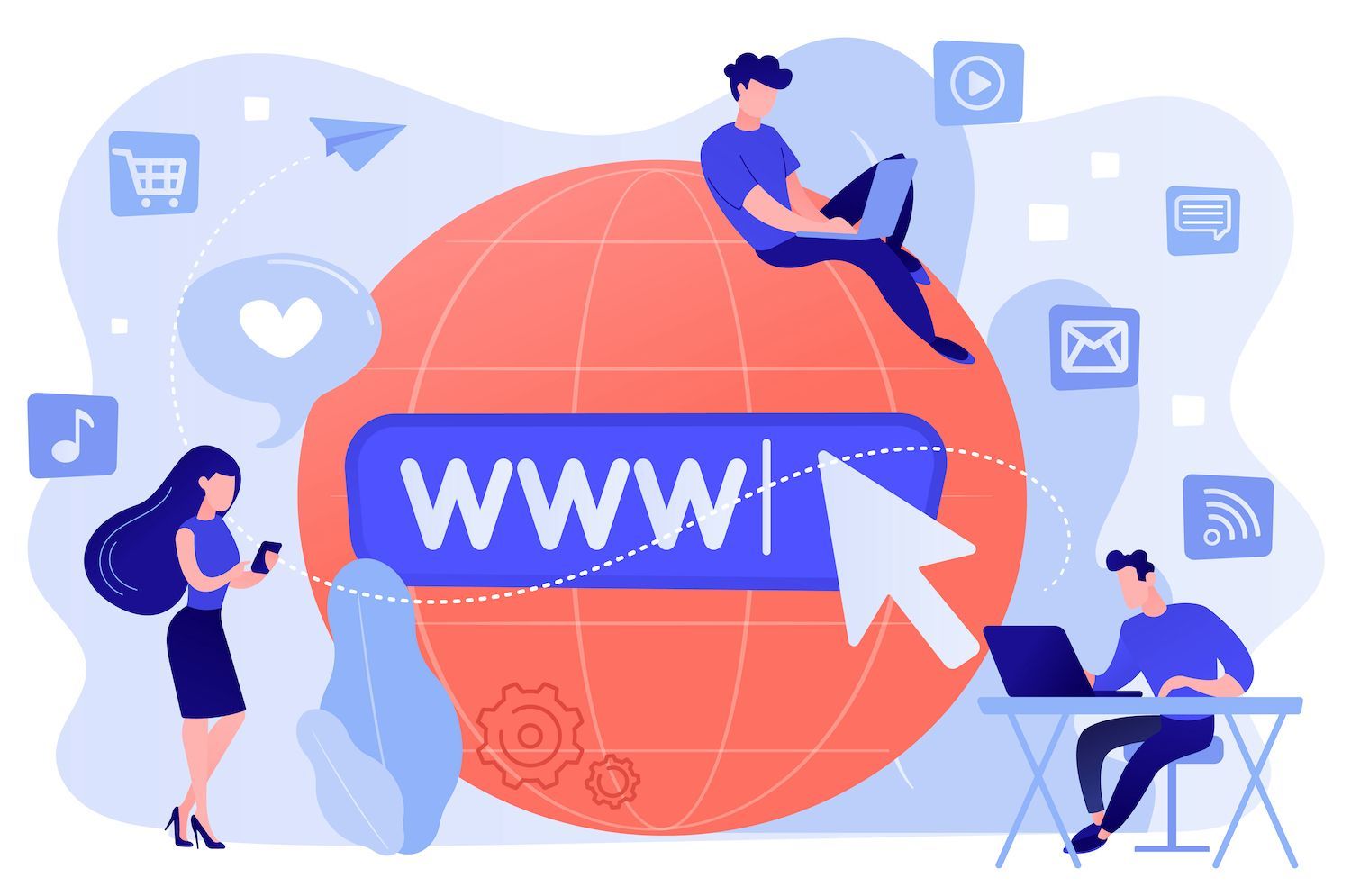
The authentic authentication function is one of web applications' most essential and basic abilities. Web frameworks like Laravel offer a variety of options for users to log in.
You can implement Laravel security features quickly and secure. But, using them in the wrong way could cause danger since malicious individuals could exploit these features.
This article can help you learn about prior to implementing your preferred Laravel authentication techniques.
Take a look!
An Intro To Laravel Authentication
The settings for authentication are stored in a file titled config/auth.php
. The file offers a variety of options to tweak and change the way that authentication is performed of Laravel.
The first step is to identify the default authentication options. The choice you choose controls the app's security "guard" and reset password choices. The default settings can to be changed as required however they're an excellent start for all apps.
Next, you define authentication guards for your application. Here, our default configuration uses the storage of sessions and Eloquent's user service. Each authentication driver comes with the user provider.
return [ /* Defining Authentication Defaults */ 'defaults' => [ 'guard' => 'web', 'passwords' => 'users', ], /* Defining Authentication Guards Supported: "session" */ 'guards' => [ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], ], /* Defining User Providers Supported: "database", "eloquent" */ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\Models\User::class, ], // 'users' => [ // 'driver' => 'database', // 'table' => 'users', // ], ], /* Defining Password Resetting */ 'passwords' => [ 'users' => [ 'provider' => 'users', 'table' => 'password_resets', 'expire' => 60, 'throttle' => 60, ], ], /* Defining Password Confirmation Timeout */ 'password_timeout' => 10800, ];
Then, we ensure that every authentication device is connected to the provider of the user. This defines how users are retrieved from your database or other methods of storage in order to protect the user's information. You can configure different sources for each model table, in the event that you have multiple models or user table. These sources may be assigned to different security guards you've specified.
Users may also want to change their passwords. In this scenario, it is possible to set different password reset parameters when you have several tables or models within your application. You may would like to apply different settings depending on a specific kind of user. The expiration period is the period during each reset token that is in use. This function makes sure that tokens last only a short time and therefore less likely to be guessed. It can be modified as required.
It is your responsibility to decide when password confirmation is timed out, and the user is required to confirm their password a second time at the time of confirmation on the screen. The timeout duration default is 3 hours.
The many kinds of Laravel Authentication methods.
A Password-Based Authentication System
A basic way to authenticate users' identities, it's widely used by thousands of companies but as technology is evolving, the process is being deemed obsolete.
Vendors are expected to implement sophisticated password implementations and ensure that users have minimal disruption.
It's quite simple it is just as easy as typing in your name and password. And, in the event that in the Database it's discovered that there's a connection between these two items, the server chooses to examine the data and let users access the resources for a specific duration of duration.
Token-based authentication
The process is used to issue the user an individual token upon confirmation.
By using this token, the user has access to the relevant information sources. The privilege is in place for a period of time until the day when the token expires.
When the token has been activated, it does not need to type in a username and password. But, once you have retrieved a fresh token it is necessary to enter both.
Tokens are frequently employed in various scenarios since they're non-stateless entities that contain all necessary authentication information.
The ability to differentiate the difference between token generation and verification lets vendors enjoy an abundance of flexibility.
Multi-Factor Authentication
The term "authority" is a reference to the idea that it should use at least two forms of authentication which will increase the amount of security that it offers.
This is generally done using an encryption technique, and then users are provided with a unique identification number to utilize on their phone. The companies that utilize this method should be aware of fake positives or network issues which could lead to serious problems when expanding quickly.
What do I need to do to Implement the Laravel Authentication
Manual Authentication
Starting with registering users and creating routes within routes/web.php
.
Two routes will be created for you to go through the application form, as well as another to sign up for:
use App\Http\Contrllers\Auth\RegisterController; use Illuminate\Support\Facades\Route; /* Web Routes Register web routes for your app's RouteServiceProvider in a group containing the "web" middleware */ Route::get('/register', [RegisterController::class], 'create']); Route::post('/register', [RegisterController::class], 'store']);
and establish the necessary control for these tasks:
php artisan make controller Auth/RegisterController -r
Make the necessary changes now by using the following method:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use illuminate\Htpp\Request; class RegisterController extends Controller public function create() return view('auth.register'); public function store(Request $request)
The controller is empty , and returns the view you are able to join. You can create the view in the views/resources/auth
and then name it register.blade.php. register.blade.php
.
After everything is done you can go on our or sign up
route, and you can then complete the form below:
public function store(Request $request) $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials, $request->filled('remember'))) $request->session()->regenerate(); return redirect()->intended('/'); return back()->withErrors([ 'email' => 'The provided credentials do not match our records. ', ]);
Then, we've got the form , which users can complete and get all of the information needed. After that, you must collect the user' information and verify the information and add it to the database to verify that all information is accurate. That's why you have make use of a transaction within your database to confirm that the data you entered is accurate.
We'll utilize Laravel's request validation tool to be certain that the three necessary credentials are available. It is imperative to ensure that your password is in line with the format and the format. Additionally, the password is unique to the users
database. The password has been verified and has the minimum amount of characters:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Foundation\Auth\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; class RegisterController extends Controller public function store(Request $request) confirmed public function create() return view('auth.register');
When our input has been verified, any input that does not conform to the validation process can result in an error. The error will be displayed as follows:
After we've set up an account on behalf of a user by using the Store
method, we'd like to login to the user's account. There are two methods for us to accomplish this. There is a way to accomplish this manually, or by using this technique using an interface called the Auth interface.
If a user logs into this system, they shouldn't go back to that Register screen. Instead, they should be directed to a different webpage, such as a homepage or the dashboard. This is how we'll accomplish this:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use App\Providers\RouteServiceProvider; use Illuminate\Foundation\Auth\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; use Illuminate\Support\Facades\Hash; class RegisterController extends Controller public function store(Request $request) confirmed public function create() return view('auth.register');
And now that we are authentic users, logged in
using the password -n, we have to make sure that the user is able to sign out safely.
Laravel suggests that we stop the session and then create an entirely new security token after the user logs out. That's exactly what we are doing. We must first come up with a new logout
procedure that uses LogoutController's method of destruction.
method:
use App\Http\Controllers\Auth\RegisterController; use App\Http\Controllers\Auth\LogoutController; use Illuminate\Support\Facades\Route; /* Web Routes Here is where you can register web routes for your application. These routes are loaded by the RrouteServiceProvider with a group which contains the "web" middleware group. Do something amazing! */ Route::get('/register', [RegisterController::class, 'create']); Route::post('/register', ['RegisterController::class, 'store']); Route::post('/logout', [Logoutcontroller::class, 'destroy']) ->middleware('auth');
The process of logging out via"auth," the "auth" middleware program is crucial. It is essential that the user cannot use this service if they're not signed in.
After that, you'll be able to create an account similar to that we had earlier:
php artisan make:controller Auth/LogoutController -r
You can ensure that the request is considered to be a part of destruction
procedure. Users are signed out with an Auth facade. After that, we disable the session after which we regenerate the token and redirect users to our home page:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class LogoutController extends Controller public function destroy(Request $request) Auth::logout(); $request->session()->invalidate(); $request->session()->regenerateToken(); return redirect('/');
Remembering Users
The majority, if not all modern websites offer that "remember me" option after you sign to their forms.
If we want to add to that "remember me" option, we could use a boolean as another argument to the"try" method.
When the token appears to be legitimate, Laravel will keep the user authenticated for an extended time or until they are manually removed. The table of users must include the string forget_token
(this is to create tokens) column. The column is where we'll save our "remember me" token.
The default migration is available to users.
To begin, add to begin,"Remember Me" to the "Remember me" field :
Then, you'll have the ability to access your credentials by completing the request and then add them to the test method of using the Auth facade.
If the user's name is available If we can identify the user's name, we'll sign him in and then redirect him to our home page. If not, we'll give an error.
public function store(Request $request) { $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials, $request->filled('remember'))) $request->session()->regenerate(); return redirect()->intended('/'); return back()->withErrors([ 'email' =>
Resetting Passwords
Most web-based apps let users reset their passwords.
Another method will be developed to reset the password that was lost and to create this controller in the exact manner as we did. Also, we'll develop the first method of reset password buttons that includes the token needed for all of the process.
Route::post('/forgot-password', [ForgotPasswordLinkController::class, 'store']); Route::post('/forgot-password/token', [ForgotPasswordController::class, 'reset']);
If you choose to store, we'll use the email address provided in the form of a request. We will verify the email address exactly the same manner as we would in normal circumstances.
Following this, we are able to implement SendResetLink as a SendResetLink
technique , accessible via the password interface.
As a result, we'd like to update the status if it succeeded in sending the link or should there be any other issue:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Password; class ForgotPasswordLinkController extends Controller public function store(Request $request) $request->validate([ 'email' => 'required
After the reset link is provided to the user's email It is our responsibility to take care of the steps that follow.
The token will be sent to you and email address together with the password change once you've sent your email address and then verify it.
Following this, we're able of trying to reset your password by using the password facade to allow Laravel manage everything else in the background.
It's always a great option to create a password hash in order to secure it.
We then verify if the password has been changed, and If it has, we send users to login screens and display it being a message "Success. If the password was not reset, we display an error that indicates that the password could not be reset.
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Password; use Illuminate\Support\Str; class ForgotPasswordController extends Controller public function store(Request $request) $request->validate([ 'token' => 'required', 'email' => 'required
Laravel Breeze
Laravel Breeze is an easy alternative to Laravel authentication capabilities, such as registration and login features for password reset, login authentication, as well as the verification of passwords. Breeze can be used to add authentication features, such as login authentication, password reset and more Breeze is a way for authentication in the new Laravel application.
Installation and installation and
After creating your Laravel application, all you need to do is to set up your database, then make the necessary migrations, and then run the app using composer.
composer require laravel/breeze -dev
Then, follow these instructions:
php artisan breeze:install
It will also publish your authentication views and routes, as well as controllers and any other information it utilizes. Once you have completed this process, you'll fully control everything Breeze offers you. Breeze offers you.
Now we need the frontend to be rendered by our application and install our JS dependencies (which make use of @vite):
Install NPM
:
npm run dev
After you've logged in following logging in the password and registration link should be displayed on your homepage and everything should work well.
Laravel Jetstream
Laravel Jetstream enhances Laravel Breeze by adding useful features and an additional stack of frontends.
It lets you sign in, register, as well as email confirmation. It also provides security with two factors along with session control. API assistance is available through Sanctum along with the administration of teams.
You can choose between Livewire or Inertia at the frontend of installing Jetstream. Backends use Laravel Fortify which is a frontend agnostic "headless" authentication backend that is used by Laravel.
Installation and Installation
We'll install it via the composer for the purposes of Laravel Project:
composer require laravel/jetstream
Then, we'll execute jetstream's jetstream install PHP jetstream[]
command, which supports stackas
options like Livewire
or Inertia
. Then, you can make use of the option of selecting the team
option to allow the feature on teams.
It will also allow you to install Pest PHP in order to testing purposes.
Then, we have to develop the front end for this application by using these techniques:
npm install npm run dev
Laravel Fortify
Laravel Fortify is a backend authentication system that is frontend-independent. It's not necessary to utilize Laravel Fortify in order to take advantage of the Laravel authentication functions.
Fortify may also be utilized to launch tools such as Breeze as well as Jetstream. Fortify runs Fortify as a standalone application, which functions as an implementation of the backend. If you choose to use Fortify as a standalone application, your frontend needs to utilize Fortify's routing routes for access to the routing routes.
Installation and Set-up
Fortify is a tool which we are able to install Fortify via composer.
composer require laravel/fortify
The next thing to do is provide Fortify's sources to the public:
php artisan vendor:publish -provider="Laravel\Fortify\FortifyServiceProvider"
After this, we will create a new app/Actions directory in addition to the new FortifyServiceProvider, configuration file, and database migrations.
Finally, run:
php artisan migrate
Or:
php artisan migrate:fresh
And then the Fortify is ready to start its journey.
Laravel Socialite
Installation
You can download it via composer:
composer require laravel/socialite
Configuration and usage
Once we have installed the application, we must connect the correct credentials to the OAuth vendor our application depends upon. The credentials will be put in config/services.php for each service.
In the configuration, you will need you verify your keys to prior services. Keys you could compare are:
- Twitter (For OAuth 1.0)
- Twitter-Oauth-2 (For OAuth 2.0)
- Github
- Gitlab
- Bitbucket
The service configuration can be in the following manner:
'google' => [ 'client_id' => env("GOOGLE_CLIENT_ID"), 'client_secret' => env("GOOGLE_CLIENT_SECRET"), 'redirect' => "http://example.com/callback-url", ],
Authenticating Users
To accomplish this you can choose between two options for redirecting users' requests to OAuth providers.
use Laravel\Socialite\Facades\Sociliate; Route::get('/auth/redirect', function () return Socialite:;driver('google')->redirect(); );
And one for the second call to the service provider after authenticating:
use Laravel\Socialite\Facades\Socialite; Route:;get('/auht/callback', function () $user = Socialite:;driver('google')->user(); // Getting the user data $user->token; );
Socialite offers the redirect method together with the facade, which redirects the user to the OAuth provider. The method for the user analyses the request before obtaining the data about the user.
When we receive the information of the user We must confirm that it is there on our database. If it's there an authentic database, we have to verify the information. If the database is not there, and there's no database available then we'll have to build an entry to represent the individual:
use App\Models\User; use Illuminate\Support\Facades\Auth; use Laravel\Socialite\Facades\Socialite; Route::get('/auth/callback', function () /* Get the user */ $googleUser = Socialite::driver('google')->user(); /* Create the user if it does not exist Update the user if it exists Check for google_id in database */ $user = User::updateOrCreate([ 'google_id' => $googleUser->id, ], [ 'name' => $googleUser->name, 'email' => $googleUser->email, 'google_token' => $googleUser->token, 'google_refresh_token' => $googleUser->refreshToken, ]); /* Authenticates the user using the Auth facade */ Auth::login($user); return redirect('/dashboard'); );
If we want to restrict the accessibility of users to certain areas, we could employ the scoping
technique. The method applies to the authentication request. This will join all of those previously mentioned areas and place them in the scope which is unique to.
Another alternative is to employ this technique: setScopes
method, which replaces any scope that is already used:
use Laravel\Socialite\Facades\Socialite; return Socialite::driver('google') ->scopes(['read:user', 'write:user', 'public_repo']) ->redirect(); return Socialite::driver('google') ->setScopes(['read:user', 'public_repo') ->redirect();
There is a lot of information regarding how to reach users via their phone numbers upon callback. It's time to glance at some of the details we could get from this.
OAuth1 user has token
as well as tokenSecret
:
$user = Socialite::driver('google')->user(); $token = $user->token; $tokenSecret = $user->tokenSecret;
OAuth2 provides you with the token
, refreshToken
and expires
:
$user = Socialite::driver('google')->user(); $token = $user->token; $refreshToken = $user->refreshToken; $expiresIn = $user->expiresIn;
Both OAuth1 as well as OAuth2 supports GetID, getName getEmail, and also getAvatar.
$user = Socialite::driver('google')->user(); $user->getId(); $user->getNickName(); $user->getName(); $user->getEmail(); $user->getAvatar();
And if we want to get user details from a token (OAuth 2) or a token and secret (OAuth 1), sanctum provides two methods for this: userFromToken
and userFromTokenAndSecret
:
use Laravel\Socialite\Facades\Socialite; $user = Socialite:;driver('google')->userFromToken($token); $user = Socialite::driver('twitter')->userFromTokenAndSecret($token, $secret);
Laravel Sanctum
Laravel Sanctum is a light authenticator ideal for SPAs (Single Page Apps) and mobile apps. It allows users to create several API tokens that have specific limits. The scope of the token defines activities that can be carried out by an API token.
Applications
Sanctum could be used to create API tokens for users without the complexities associated with OAuth. These tokens usually have lengthy time frames, similar to the years. They are however easily changed and can be cancelled by clients at any time.
Installation and installation as well as
It's possible to set it up using composer
composer require laravel/sanctum
It is also essential to create the files to allow for migration and configuration:
php artisan vendor:publish -provider="Laravel\Sanctum\SanctumServiceProvider"
After we've created new documents for the migration and are in the process of preparing to transfer the following files:
php artisan migrate or php artisan migrate:fresh
What's the most efficient way to issue API tokens?
Before issuing tokens, our User model should use the Laravel\Sanctum\HasApiTokens trait:
use Laravel\Sanctum\HasApiTokens; class User extends Authenticable use HasApiTokens;
When we have the user, we can issue a token by calling the createToken
method, which returns a Laravel\Sanctum\NewAccessToken instance.
You can use PlainTextToken as a PlainTextToken
method within the NewAccessToken instance to display the SHA-256 the text in its simple shape within the token.
Strategies and the best methods for authenticating Laravel
Validating Sessions across Different Devices
We have previously discussed it is essential after the user logs off. The session, however, should be accessible on every device belonging to the person.
This is usually used in situations where the user alters or alters their password, in order to remove their account on other devices.
Making use of the Auth facade, it's an easy process. Because the method being used comes along with auth
together with auth.session middleware auth.session middleware
We can use this method to shut down the other devices
with the static approach that is provided by the facade
Route::get('/logout', [LogoutController::class, 'invoke']) ->middleware(['auth', 'auth.session']);
use Illuminate\Support\Facades\Auth; Auth::logoutOtherDevices($password);
Configuration With Author Routes()
The route method in the Auth facade functions as a spokesman to produce the necessary routes to facilitate authentication of users.
The choices are The options are Login (Get Post or Search), Logout (Post) or Register (Get Post or Get) and Password Change/Email (Get Post and Get).
If you can transfer the process to the façade, this can result in:
public static fucntion routes(array $options = []) if (!static::$app->providerIsLoaded(UiServiceProvider::class)) throw new RuntimeException('In order to use the Auth:;routes() method, please install the laravel/ui package. '); static::$app->make('router')->auth($options);
We'd like to know how we can use the static method called on the router. This is a tricky job because of how facades function however the technique used is like the following:
// >/** Sign up for the most common methods of authentication for applications. @param array $options @return void */ public function auth(array $options = []) // Authentication Routes... $this->get('login', 'Auth\[email protected]')->name('login'); $this->post('login', 'Auth\[email protected]'); $this->post('logout', 'Auth\[email protected]')->name('logout'); // Registration Routes... if ($options['register'] ?? true) $this->get('register', 'Auth\[email protected]')->name('register'); $this->post('register', 'Auth\[email protected]'); // Password Reset Routes... if ($options['reset'] ?? true) $this->resetPassword(); // Email Verification Routes... if ($options['verify'] ?? false) $this->emailVerification();
It will generate by default all routes, with the exception of it from the email authentication route. It generates both Login as well as Logout routes. We can also control the other routes. choose to control using the selection option.
If we want to permit users to sign in and leave but not sign in, then we can use these arrays of options:
$options = ["register" => true, "reset" => false, "verify" => false];
Security Routes, Custom Guards, and Protecting Routes
We need to ensure that the routes we utilize can be accessible only for authenticated users. This is simple to do this either by using the middleware method at the front of Route or by using the middleware method in the setting of the middleware method.
Route::middleware('auth')->get('/user', function (Request $request) return $request->user(); ); Route::get('/user', function (Request $request) return $request->user(); )->middleware('auth');
This security measure ensures every request received is authenticated.
Password Confirmation
To ensure website security You will often need to verify the password for the individual prior to moving on to the next position.
It is crucial to choose an appropriate path starting through the viewpoint of confirm password, in order to complete the request. The system will confirm and then send the user to their final destination. We also ensure that the password that we choose to use is validated during the duration of the session. In default, the password is verified every three hours. It is possible to alter the frequency through modifying the configuration file in config/auth.php:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Redirect; Route::post('/confirm-password', function (Request $request) if (!Hash::check($request->password, $request->user()->password)) return back()->withErrors([ 'password' => ['The provided password does not match our records.'] ]); $request->session()->passwordConfirmed(); return redirect()->intended(); )->middleware(['auth']);
Authenticable Contract
The Authenticable contract located at Illuminate\Contracts\Auth defines a blueprint of what the UserProvider facade should implement:
namespace Illuminate\Contracts\Auth; interface Authenticable public function getAuthIdentifierName(); public function getAuthIdentifier(); public function getAuthPassord(); public function getRememberToken(); public function setRememberToken($value); public function getrememberTokenName();
The interface allows the authentication system to work with every "user" class that implements it.
This is true regardless of the ORM or storage layer employed. In the default Laravel includes the app Models User that implements this interface. It can be seen within the configuration files:
return [ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\Models\User::class, ], ], ];
Authentication Events
Many events that happen during the process of authentication.
Depending on your goals, you can attach listeners to those events in your EventServiceProvider
.
Rapidly create new users
A process for creating a new user quickly can be achieved by the app user:
$user = new App\User(); $user->password = Hash::make('strong_password'); $user->email = '[email protected]'; $user->name = 'Username'; $user->save();
either through or using the static creation method that is available or through or through the interface for the user:
User::create([ 'password' => Hash::make('strong-password'), 'email' => '[email protected]', 'name' => 'username' ]);
Summary
The Laravel ecosystem comes with a range of kits that can be used to make the application running, which utilize an authentication system, like Breeze as well as Jetstream. The kits are highly customizable as the code is created by us as well as we are able to modify the method we'd prefer to employ the blueprint if it be necessary to.
There are many security concerns regarding authentication and the difficulties associated with it. Each of these issues can be addressed easily making use of the tools Laravel can provide. They can be easily customizable and simple to use.
- Simple setup and management and management My Dashboard. My dashboard
- Support is available 24/7.
- Most efficient Google Cloud Platform hardware and network are powered by Kubernetes to provide the most capacity
- An enterprise-level Cloudflare integration that improves efficiency and security.
- Global reach with as many as 35 data centers and over 275 PoPs distributed around the globe
Article was posted on this website
This post was posted on here